Writing Custom Policies
This article is subject to change as the feature develops and we make improvements.
In this article we'll cover the main components of what's needed when writing a policy. To help with this, we'll be referencing the Monitor Authentications (MA) policy template that's available in HYPR Adapt. This template was built specifically to have the basic functionality needed to have a working Adapt policy.
Prerequisites
Writing an Adapt policy first requires knowledge in the language used to write the policies. Policies are written in a declarative language called Rego. The benefit of this language is that it makes reading and writing policy code easier. Before going through this guide, we recommend that you look through the documentation for Rego so that you have better context of what's happening in your policy code.
Overview
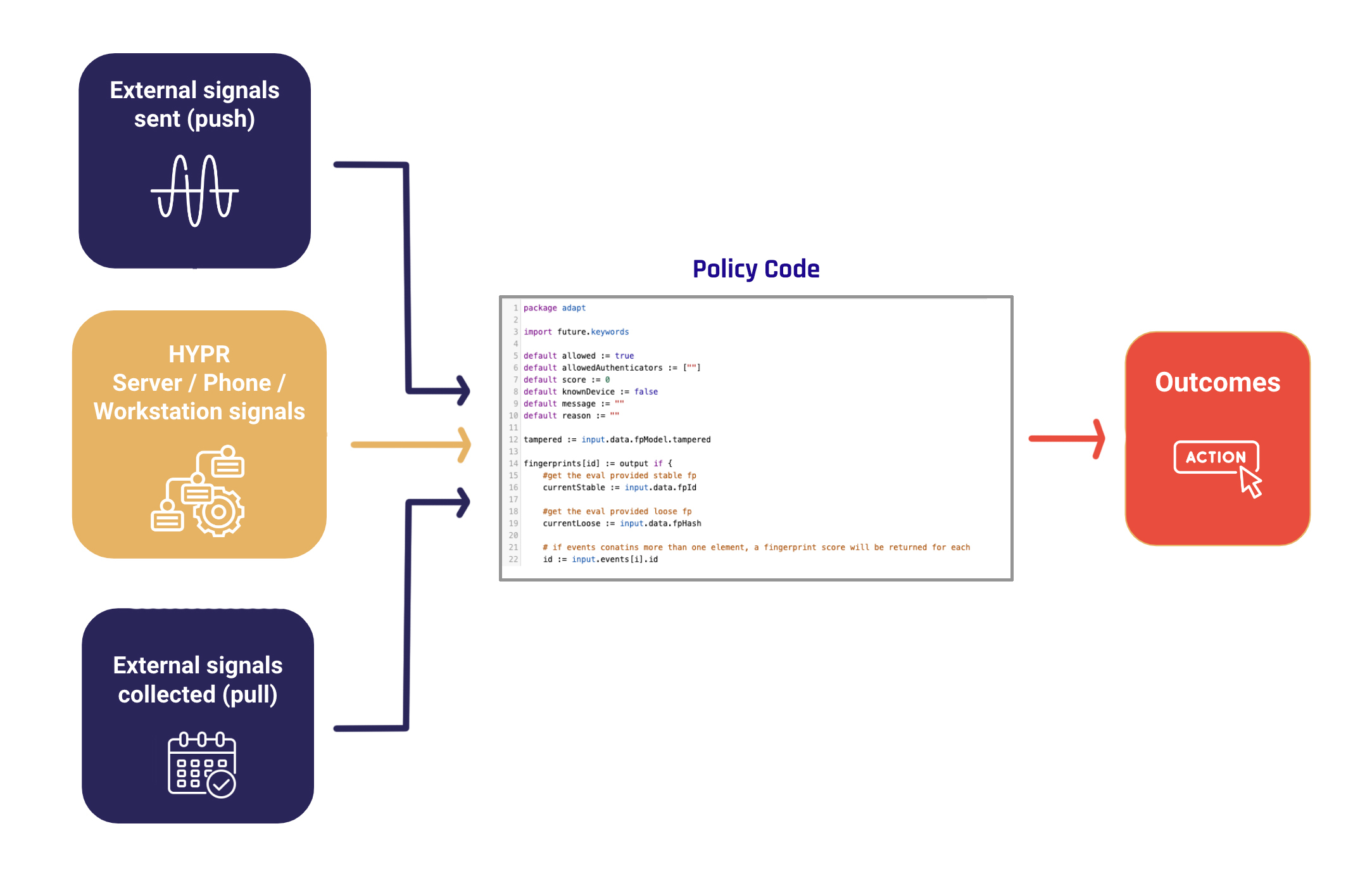
A policy is always evaluated for an entity. An entity may be a user, phone, workstation, or browser.
When the policy is evaluated, the system gathers all available Events for the entity and passes them to the policy code.
The output from the policy code dictates the authentication outcome or risk posture.
Policy Code Components
Packages
package authz
The first line of code in the MA policy is the package declaration. The package defines the namespace for your policy rules. Any name is valid, but for Adapt policies we typically use package authz
. The package declaration is required for the policy to run.
Imports
import future.keywords.in
import future.keywords.if
Open Policy Agent (OPA) has keywords that have been introduced since the initial release. If you want to use these keywords, you must import them as shown in the image above. See OPA Future Keywords for a list of available future keywords.
Common keywords that may be helpful are if
and in
(as shown above). These keywords help make a policy rule easier to comprehend, or provide a shorthand way to write common logic. They're not required for your policy, but they help make policies easier to write.
You can also add imports for your policy input. For example, if you need a shorthand way to refer to a list of Events stored in your policy input, instead of typing input.events
everywhere it's needed, you can instead use import input.events
or import input.events as events
. Either option will let you reference input.events
with just events
.
Documents and Rules
Policy decisions are made by the policy engine receiving some input; using that input to determine the result of certain defined values; and returning those values in the evaluation result. We can refer to these values as documents and the logic used to determine their result as rules. Documents can take the form of any type of value: Booleans, numbers, strings, objects, arrays, etc. With all this said, when you go further down the code you will see this:
default allowed := true
default allowedAuthenticators := []
default message := "Policy evaluation successful"
blockOnFailedEvaluation := %%block_on_failed_eval%%
Here we use the default
keyword to set the default values of some documents that we want returned in our evaluation result. Though setting default values isn't required, we do this here because the documents listed must be returned by an Adapt policy for the evaluation to succeed. Even if some are not needed, we must have a value set for them somewhere in our policy code. In the MA policy we use defaults; if any later rules return undefined
, we have a fallback value in place to return. So it's recommended to have a default value set for the documents above before writing your rules.
Here's what these documents can be used for:
-
allowed
- This is commonly used to determine if the user's action will be "allowed" to go through. It is usually applicable when a user tries to login. The final result of this value should either betrue
orfalse
. -
allowedAuthenticators
- If your policy is evaluating an authentication attempt, this value can be used to set which authenticators can still be used when the allowed value is set tofalse
. This will be in the form of an array of strings whose values represent an authenticator (e.g.,QR
= QR authentication). If your policy doesn't need allowed authenticators, then this can be set to an empty array as shown above. -
message
- User-friendly text that the policy can send back explaining the result of an evaluation. The result of this value will most likely be based on the computed values ofallowed
orallowedAuthenticators
.
We also define a blockOnFailedEvaluation
document that pulls data from our policy configuration. This isn't required for our policy, so we choose not to set a default. This will be used for readability purposes.
Global Input Document
As mentioned earlier, your policy will set document values using rules; those rules will generate a value based on input provided for them. This input is provided using the global input document that gets exposed by the policy engine.
In Adapt, we attach two attributes to the global input document:
-
events
- This is an array of all the Events (within a certain time period) available for the entity. These maye be HYPR Events or Events from an external ecosystem (such as user's location, IP address, etc). It is likely that your policy decision making will be based on what is included in these Events. This attribute can be accessed by usinginput.events
in the policy code. -
data
- This will include any additional information:-
workstationSignal
- Present if signal data is sent by the HYPR Passwordless application, inline with the current authentication request. Inline signals are opt-in and must be enabled via feature flags. This attribute can be accessed by usinginput.data.workstationSignal
. -
deviceSignal
- Present if signal data is sent by the HYPR Mobile App, inline with the current authentication request. Inline signals are opt-in and must be enabled via feature flags. This attribute can be accessed by usinginput.data.deviceSignal
. -
browserSignal
- Present if signal data is sent by the Browser, inline with the current authentication request. Inline signals are opt-in and must be enabled via feature flags. This attribute can be accessed by usinginput.data.browserSignal
. -
The
data
will also contain extra attributes passed in on thedata
attribute of the Policy Evaluation API request. -
httpRequest
(Since version 9.5) attribute containing details on http request bring processed for Authentication. Always present. Can be accessed by usinginput.data.httpRequest
. It contains the following attributes:method
uri
headers
queryString
remoteIP
-
evaluationRequest
(Since version 9.5) for the policy evaluation. Always present. This attribute can be accessed by usinginput.data.evaluationRequest
. Contains the following attributes:tenantUuid
of current tenantpolicyId
of the policy being evaluateduserName
of the user or entity the policy is being evaluated forsupportingEntities
additional users or entities relevant to this policy evaluation
-
requestContext
(Since version 9.5) containing any extra information available in the authentication context when the HYPR CC requests policy evaluation at the assigned evaluation point.
-
Both attributes can be accessed by using input.events
and input.data
, respectively. Most if not all your rules will require accessing these attributes.
While in test mode, you can inspect these by simply printing them out from the policy
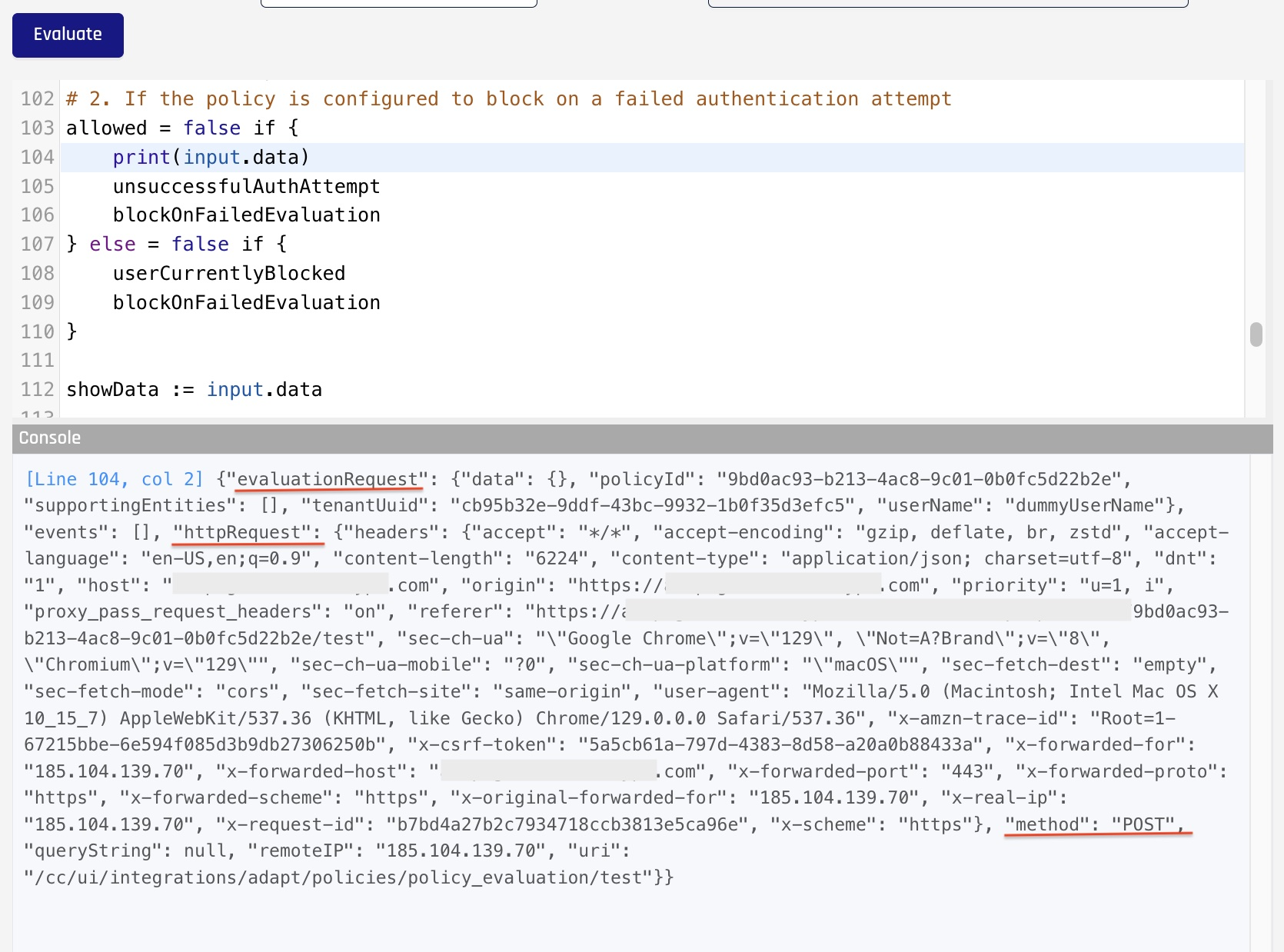
Please contact HYPR Support to enable any desired feature flags.
Rule Definitions
Going down further we see our first rule definition:
unsuccessfulAuthAttempt if {
some event in array.reverse(input.events)
isAuthEvent(event)
withinTimeframe(event)
event.isSuccessful == false
}
Rules essentially define a list of conditions that must be met for a document to be assigned a certain value. If you don't specify value to be assigned, it defaults to true
. In other words, this block of code can also be written like this:
unsuccessfulAuthAttempt := true if {
some event in array.reverse(input.events)
isAuthEvent(event)
withinTimeframe(event)
event.isSuccessful == false
}
Also notice the use of if
and in
for our rule. We can omit if
and the code below will still be valid, but the code is a little easier to understand when it is used:
unsuccessfulAuthAttempt {
some event in array.reverse(input.events)
isAuthEvent(event)
withinTimeframe(event)
event.isSuccessful == false
}
Using in
allows us to loop through Events in the input and contain the logic for processing each item within the rule.
Going back to the rule definition, this rule does the following:
-
unsuccessfulAuthAttempt
should equaltrue
if:-
Some (at least one) Event(s) in a list of Events:
-
Is an authentication Event (using a function which we will talk about later) AND
-
Occurs within a certain timeframe AND
-
Was successful (through the
isSuccessful
attribute)
-
-
Since we didn't define a default value, unsuccessfulAuthAttempt
will only be returned if it results to true
. If you want it to be included in your final result, you can either set a default value or create a second rule where it should evaluate to false
.
You can use the result of one rule to set the result of another rule. Below are two other rules we have defined in the MA policy that reference unsuccessfulAuthAttempt
:
allowed = false if {
unsuccessfulAuthAttempt
blockOnFailedEvaluation
} else = false if {
userCurrentlyBlocked
blockOnFailedEvaluation
}
message = "Failed authentication event has occured" if {
unsuccessfulAuthAttempt
not userCurrentlyBlocked
not blockOnFailedEvaluation
} else = "Failed authentication event has occured. This user will now be blocked." if {
unsuccessfulAuthAttempt
not userCurrentlyBlocked
blockOnFailedEvaluation
} else = "This user is currently blocked" if {
userCurrentlyBlocked
blockOnFailedEvaluation
}
You can also chain rules with else
if you have multiple rules for the same document and want more control over the flow order of the rules. The document will equal the first rule that matches and ignore the remaining rules in the chain.
We have userCurrentlyBlocked
referenced here as well. Refer to the Including Action Events section for a full description.
These rules will override the respective default values that we defined earlier only if all the conditions are met.
The rules we have defined in our MA policy are what's known as complete rules; each document can only equal one value. If you have two different rules for the same document and both rules evaluate to the same value, your policy evaluation will fail with an error.
To avoid this, try keeping the rule conditions unique between two rules, or use else
to chain rules for a single document.
Functions
In our unsuccessfulAuthAttempt
rule, we use two functions to help set the rule conditions:
isAuthEvent(event)
withinTimeframe(event)
Use functions to contain shared logic or as a separation of concerns. Functions will also help make your policy rules more readable. Below are the definitions for the functions mentioned above:
isAuthEvent(event) {
authEvents := ["OOB_WEBSITE_AUTH", "SESSION_WEBSITE_AUTH", "FIDO2_WEBAUTHN", "SMARTKEY_AUTH"]
event.eventName in authEvents
}
withinTimeframe(event) {
currentTimeInMilli := time.now_ns()/1000000
eventTime := to_number(event.eventTimeInUTC)
timePeriodInMilli := %%time_period%% * 60 * 1000
currentTimeInMilli - eventTime < timePeriodInMilli
}
The first function verifies whether or not the Event name is equal to any of the Event names we want to check.
The second function takes the timestamp of an Event and confirms if that timestamp is within the time window we have set in our policy configuration.
Continue learning about HYPR Adapt Policies in Including Action Events.