Saving Events from a Policy
This article is subject to change as the feature develops and we make improvements.
The policy may pull some Events and then want to look up information on an entity in the Events. For example:
-
The policy is given a workstation Event with the CrowdStrike ID on it; now it will try to search all Events on the CrowdStrike ID
-
An evaluation request comes in with an IP address in the header; the policy now wants to search Events on the IP address
-In some scenarios the policy may need to persist a piece of data:
-
The policy made an API call and wants to persist the retrieved data for future reference.
-
The policy acquired a temporary token from OAuth; and wants to save this token for short term use between separate invocations
To handle these scenarios, the policy rego code is allowed to make the following calls to the Adapt service:
-
Searching for Adapt Events
-
Saving Events to Adapt
Overview
Adapt Lambda exposes a local web service which enables the policy to make HTTP API calls to it.
This avoids the need to make external network calls while retaining the ability to search arbitrary event data.
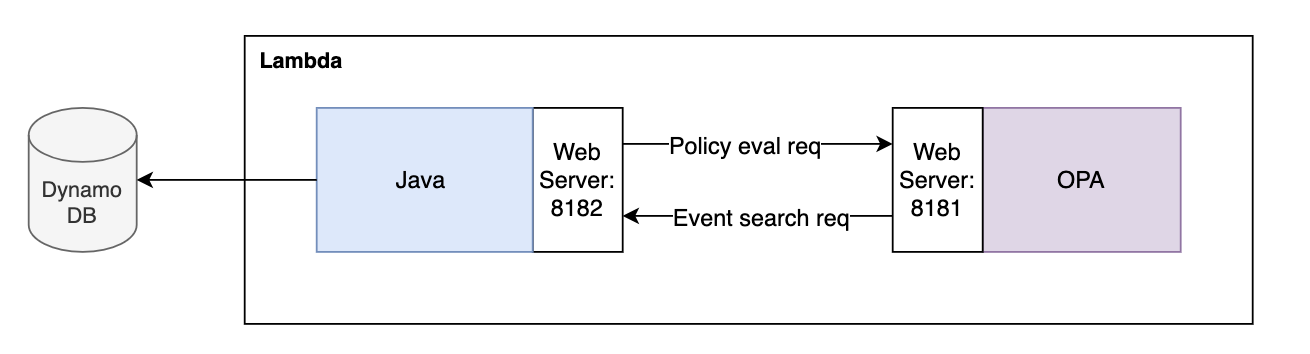
API Security
Concerns
Activity is limited to the current tenant; i.e., policies are unable to request or save data for a different tenant
Implementation
Adapt generates a short-lived (duration of the Lambda), tenant-specific API token and passes it to the policy as the input.data.securityToken
attribute.
The http_send
call sends this back in the body.securityToken
attribute. Since the Lambda only knows the token for its current tenant, it cannot lookup cross-tenant data.
Event Search API
Adapt policies use the built-in rego http.send
to make an API call the local web server.
Request Attributes
-
securityToken
[mandatory] : Security token to replay back to the search API. This associates the tenant in this policy to the subsequent search request. Ensures that the call is secure and pinned to the tenant. -
entityIdOrName
[mandatory] : Entity will typically be pulled out of the input -
startDate
[optional] : Start date for this search. Keep search window small to avoid performance issuesSample policy code; default value:7 days ago
-
endDate
[optional] : End date for this search request; default value: Date.now() -
sortKeyOrderBy
[optional] : Sort order for the sort key [ASC
|DESC
]; default value:ASC
What Is a Sort Key?Adapt uses a composite primary key, this type of key is composed of two attributes:
-
The first attribute is the partition key
-
The second attribute is the sort key
A table has only one partition key, and no two items can have the same partition key value.
Adapt uses
entityIdOrName
as the partition key. Since we have duplicates here, we use the sort key so the combination stays unique. -
-
limit
[optional] : Number or records to return; for example, to get the latest record, sort byDESC
and set a limit of1
. Default value:all records
Event Save API
Policy uses the built-in rego http.send
to make an API call the local web server.
Request Attributes
-
securityToken
[mandatory] : Security token to replay back to the search API. This associates the tenant in this policy to the subsequent search request. Ensures that the call is secure and pinned to the tenant -
eventSource
[mandatory] : Source of this Event; example:CROWDSTRIKE
-
eventName
[mandatory] : Name for this Event -
entityIdOrName
[mandatory] : Name for the entity being represented by this Event -
entitySortKey
[optional] : Custom sortKey; typically not required; default: Current timestamp in epoch -
expireInSecs
[optional] : Expiry time in seconds from now; expiration is not guaranteed to be at the exact time, but will happen eventually; default:5 years
-
event
[mandatory] : Object representing the Event data to be saved -
disableLogging
[optional] : Do not generate logs for this request processing; performance optimization for high volume situations; default:true
Sample Policy
package authz
import future.keywords.if
import future.keywords.in
default allowed := true
default allowedAuthenticators := []
default message := "Policy evaluation successful"
# Get the current time in milliseconds
current_time_millis := time.now_ns() / 1000000
# Get the time one day ago in milliseconds
one_day_ago_millis := (time.now_ns() - (24 * 60 * 60 * 1000000000)) / 1000000
# Function to search for an existing token event
search_token_event(token_name) = response {
print("Searching for saved token: ", token_name)
# Make a local call to the risk engine, to search for an event
# At times, we do not know the entity we need for evaluating the policy upfront
# In these situations, the local search may be used
# NOTE: Use it sparingly, performance overhead
http_response := http.send({
"method": "POST",
"url": "http://localhost:8182/local_search_events",
"headers": {"Content-Type": "application/json"},
"body": {
"securityToken": input.data.securityToken,
"entityIdOrName": token_name,
"startDate": one_day_ago_millis,
"endDate": current_time_millis,
"sortKeyOrderBy": "DESC",
"limit": 1
}
})
response := http_response.body
}
# Function to save a new token (as an event)
save_token_event(token_name, token_value) = response {
print("Saving new token: ", token_name, token_value)
# Make a local call to the risk engine to save an event
# This is just like saving any other event
# Only difference is that we are doing it from the policy
# NOTE: Use it sparingly, performance overhead
http_response := http.send({
"method": "POST",
"url": "http://localhost:8182/local_save_event",
"headers": {"Content-Type": "application/json"},
"body": {
"securityToken": input.data.securityToken,
"eventSource": token_name,
"eventName": "GENERATED_NEW_TOKEN",
"entityIdOrName": token_name,
"event": { "tokenValue": token_value, "createdAt": time.now_ns()/1000000 }
}
})
response := http_response.body
print("Save results: ", response)
response
}
# In this example, the policy gets a token which is valid for 10 secs
# Only after 10 secs have passed, we want to get a new token
# So the policy saves the token for 10 secs via a local save call
getToken(token_name) := token_value {
saved := search_token_event(token_name)
count(saved) > 0
print("Saved token exists")
not isExpired(saved)
print("Is not expired")
token_value := saved.tokeValue
} else := token_value {
# If no saved token found, generate and save a new token
# Create a random token based on time
token_value := sprintf("token-%d", [rand.intn("def", 1000000)])
# Save the new token and return its value
saved := save_token_event(token_name, token_value)
}
isExpired(saved) if {
millisSinceCreation := to_number(time.now_ns()/1000000) - to_number(saved[0].createdAt)
print("millisSinceCreation =",round(to_number(millisSinceCreation)))
isExpired := millisSinceCreation > 5000
isExpired
}
token := getToken("my-api-token")
Policy Test Data
[
{
"machineUserName": "baljeet.sandhu@hypr.com",
"tenantUuid": "91b90c71-50c2-4945-8d40-6e03e8160a36",
"serverRelVersion": "9.6.0-SNAPSHOT",
"remoteIP": "24.46.82.128",
"origin": "localregion",
"eventTimeInUTC": "1726667843209",
"source": "CC"
}
]
Response
The data from the search is captured in the httpResponse.body
.
If the search does not find any data, the httpResponse.body
attribute will be an empty array.
{
"message": "Policy evaluation successful",
"allowed": true,
"allowedAuthenticators": [],
"result": {
"allowed": true,
"allowedAuthenticators": [],
"current_time_millis": 1730233214912000,
"message": "Policy evaluation successful",
"one_day_ago_millis": 1730146814912000,
"token": "token-768685"
},
"policyId": "",
"policyName": "",
"policyVersion": "",
"evalDataStartDate": 0,
"evalDataEndDate": 0,
"evalEventCount": 0,
"lambdaRequestId": "",
"traceId": ""
}
Control Center UI
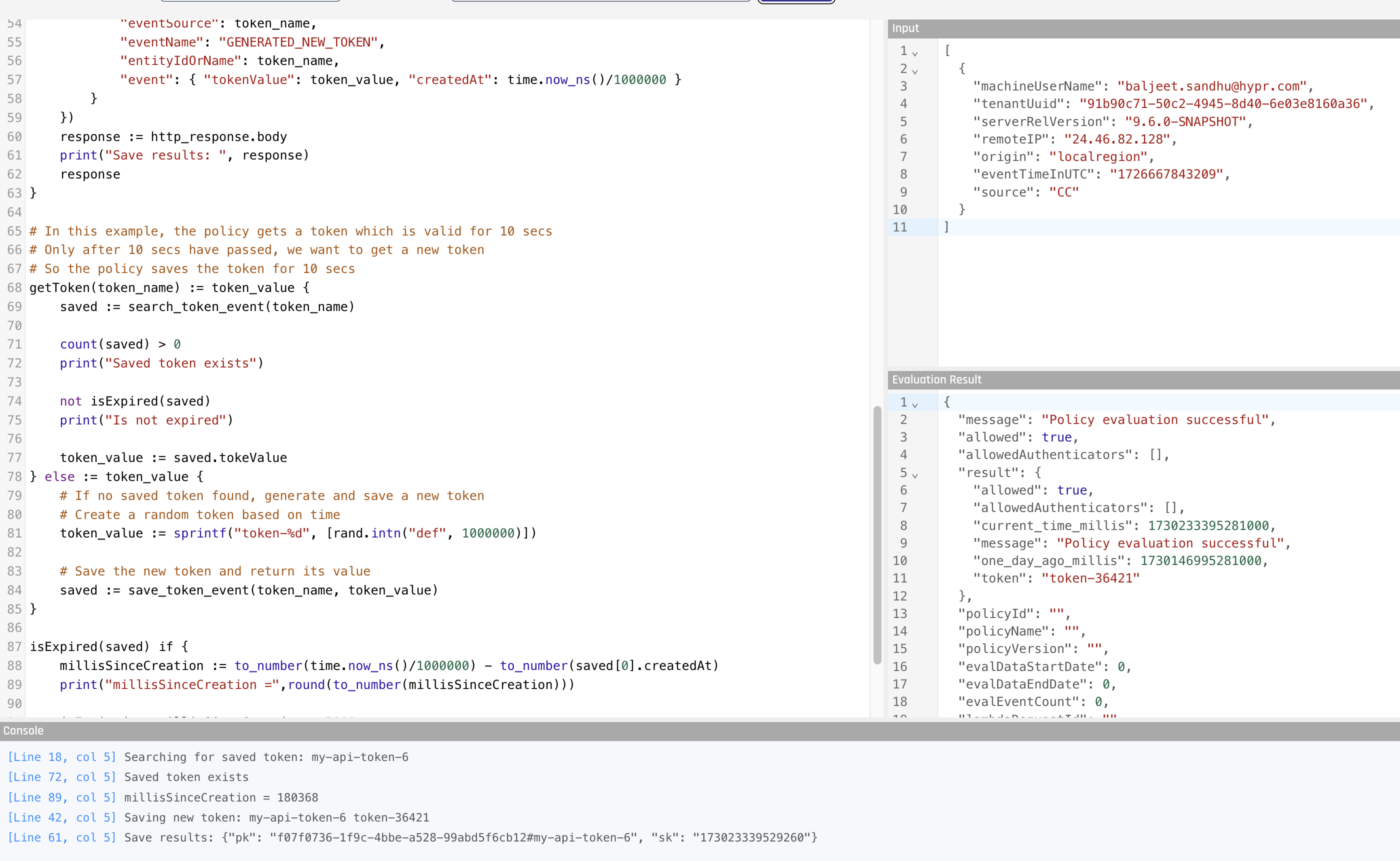